C Optimizations
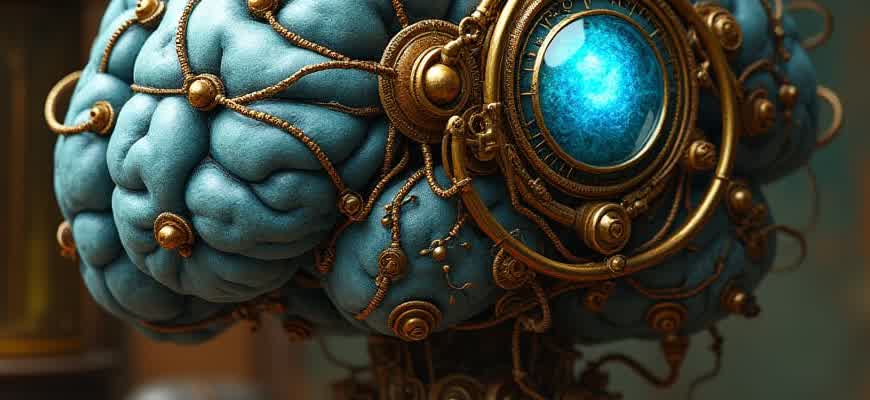
Optimizing C code is essential for improving the performance and efficiency of software. Several techniques can be used to reduce execution time, memory usage, and improve overall system responsiveness. Below are some common optimization strategies:
- Loop Optimizations: Adjusting loop structures and minimizing redundant calculations can lead to significant improvements.
- Memory Management: Efficient memory allocation and deallocation minimize overhead and prevent memory leaks.
- Compiler Optimizations: Leveraging compiler flags and settings to improve code generation can enhance performance without manual code adjustments.
Some of the key practices for C optimizations include:
- Inlining functions to avoid the overhead of function calls.
- Reducing the use of global variables to limit unnecessary memory access.
- Using bitwise operations where applicable to speed up computations.
"Optimizing code often involves trade-offs between readability and performance. It’s important to assess the impact of optimizations on maintainability."
In the table below, we compare the performance of various optimization techniques:
Optimization Technique | Performance Gain | Complexity |
---|---|---|
Loop Unrolling | High | Moderate |
Function Inlining | Moderate | Low |
Memory Pool Allocation | High | High |
Boosting Processing Speed: Practical Methods for Optimizing Data Handling in C
Efficient data processing is a critical aspect of performance optimization in C programming. With the increasing demand for real-time systems and large-scale data handling, fine-tuning code can result in significant performance improvements. In this context, various strategies can be applied to reduce execution time and memory usage, making programs more responsive and scalable.
This article explores practical techniques that can help developers achieve faster data processing through effective optimizations. These methods span from leveraging low-level features of C to utilizing algorithmic changes, both of which play a vital role in enhancing performance across different types of applications.
Key Techniques for Speed Enhancement
- Data Locality Optimization: Ensure that frequently accessed data resides close in memory to minimize cache misses.
- Efficient Memory Usage: Minimize memory allocations and use stack memory where possible to reduce overhead.
- Loop Unrolling: Unroll loops to decrease the number of iterations and increase parallelism.
- Increased Use of Bitwise Operations: Employ bitwise shifts and masks for mathematical calculations, offering faster execution compared to arithmetic operations.
Optimizing Loops and Conditional Statements
- Minimize Branching: Avoid unnecessary branches in hot code paths by simplifying conditional logic.
- Prefetching Data: Use prefetch instructions to load data into the cache before it’s needed, reducing waiting time during processing.
- Use Compiler Intrinsics: Leverage platform-specific compiler intrinsics for more control over hardware operations.
Comparison of Memory Access Patterns
Access Pattern | Impact on Performance |
---|---|
Sequential Access | Faster cache utilization and reduced latency |
Random Access | Increased cache misses and slower execution |
"When optimizing performance, always remember that the low-level details, such as memory access patterns, are just as important as the algorithm itself."
Leveraging Parallelism: Optimizing C Code for Multi-Core Processors
Modern processors feature multiple cores, enabling simultaneous execution of tasks. Optimizing C code to take full advantage of this multi-core architecture can significantly improve performance, especially for compute-intensive applications. However, efficiently distributing workloads across cores requires careful consideration of both parallel algorithms and thread management.
To achieve parallelism in C, developers need to design code that divides tasks into smaller, independent units of work. These tasks can then be executed concurrently on different cores. Utilizing libraries like OpenMP, Pthreads, or even low-level processor-specific intrinsics allows the developer to take control of thread management and task scheduling.
Key Approaches to Parallelism
- Thread-based Parallelism: Dividing a problem into smaller threads that can be executed concurrently on different cores. Thread libraries such as Pthreads or OpenMP can be used to manage the threading process.
- Data Parallelism: Splitting large datasets into chunks and processing each chunk in parallel. This is often used in applications such as image processing or scientific simulations.
- Task Parallelism: Identifying independent tasks in a program that can be run in parallel. Each task operates on different parts of the problem without dependencies.
Optimization Considerations
Efficient memory usage and minimizing synchronization overhead are critical factors in multi-core optimization. Over-using locks or excessive thread synchronization can result in performance degradation due to thread contention.
- Minimize Thread Contention: Reduce the number of threads competing for the same resources, as thread contention can lead to significant overhead.
- Data Locality: Ensure that threads access memory that is local to their execution context. This minimizes cache misses and memory latency.
- Load Balancing: Distribute tasks evenly among threads to avoid some cores being idle while others are overloaded.
Example: Parallelizing a Simple Task
Task | Single-core Execution | Multi-core Execution |
---|---|---|
Sum of Elements | Sequential iteration over an array to calculate the sum. | Divide the array into segments, each processed by a different core, then combine results. |
By carefully applying parallelism strategies, developers can maximize the utilization of multi-core processors, ultimately leading to faster and more efficient programs. However, understanding the challenges of thread synchronization and memory access is key to achieving the desired performance gains.
Optimizing Algorithms in C: From Theory to Real-World Performance
Optimizing algorithms in C is a critical part of improving software performance, especially for applications that demand high efficiency, such as embedded systems or real-time computing. Theoretical analysis provides valuable insights into the complexity of algorithms, but translating these theoretical optimizations into actual code improvements is where real-world challenges arise. Performance enhancements are not just about faster execution times, but also minimizing memory usage, maximizing processor utilization, and reducing input/output (I/O) overhead.
In practice, achieving optimal performance often involves a mix of algorithmic improvements and low-level optimizations tailored to the hardware and the specific runtime environment. From algorithmic changes like reducing time complexity to hardware-aware tweaks like loop unrolling and memory alignment, the path to efficiency requires a combination of theory, empirical testing, and a deep understanding of how the C compiler translates code into machine instructions.
Key Strategies for Optimizing Algorithms
- Choosing the Right Data Structures: A poorly selected data structure can drastically increase the computational cost. For example, using a linked list where a hash table would suffice can result in significantly slower performance.
- Minimizing Memory Allocations: Frequent dynamic memory allocation and deallocation can cause fragmentation and slow down performance. Allocating memory in larger blocks or reusing memory buffers can help mitigate these issues.
- Reducing Algorithmic Complexity: Optimizing algorithms by reducing time complexity (e.g., from O(n²) to O(n log n)) can have a profound impact on performance for large datasets.
Real-World Performance Considerations
When optimizing in C, it's essential to focus on the specific characteristics of the target environment. For instance, certain algorithms might perform differently depending on the cache hierarchy of the processor or the size of the dataset. Optimizations that work well in theory may fail to deliver real-world gains if they are not aligned with hardware properties.
Important: Profiling tools such as gprof and Valgrind are invaluable for identifying performance bottlenecks and memory issues before proceeding with optimizations.
- Optimize frequently executed paths: Focus on hot spots in the code, those that are executed most often, as they provide the highest return on investment in terms of performance improvement.
- Consider hardware-specific optimizations: Tailor your code to leverage processor-specific instructions, such as SIMD (Single Instruction, Multiple Data), when possible.
- Use compiler optimization flags: Compilers like GCC provide various flags (e.g., -O2, -O3) that can automatically apply optimizations like inlining functions, loop optimizations, and dead code elimination.
Performance Comparison Table
Optimization Method | Typical Performance Gain | Application |
---|---|---|
Algorithm Complexity Reduction | Up to 10x or more | Sorting, Searching, Graph Algorithms |
Loop Unrolling | 20%-30% speedup | Numerical Computation |
Memory Pooling | 5%-50% improvement | Embedded Systems, Game Engines |